c:choose
, c:when
, c:otherwise
タグを使うと JSP 内で条件分岐が行えます。
c:choose
, c:when
, c:otherwise
は組み合わせて使います。
目次
c:choose, c:otherwise のパラメータ
なし
c:when のパラメータ
属性 | 必須 | 意味 |
---|---|---|
test | yes | タグで囲んだ部分を表示するかの条件 |
サンプルコード
プロジェクト構成
サンプルコードのプロジェクトは以下のファイルから成ります。
- pom.xml
- src/main/java/com/example/ExampleServlet.java
- src/main/webapp/WEB-INF/jsp/index.jsp
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>example</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>war</packaging>
<name>example</name>
<build>
<plugins>
<plugin>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-maven-plugin</artifactId>
<version>9.4.31.v20200723</version>
</plugin>
</plugins>
</build>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
src/main/java/com/example/ExampleServlet.java
package com.example;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/")
public class ExampleServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setAttribute("num", req.getParameter("num"));
RequestDispatcher dispatcher = req.getRequestDispatcher("/WEB-INF/jsp/index.jsp");
dispatcher.forward(req, resp);
}
}
src/main/webapp/WEB-INF/jsp/index.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head>
<title>Test</title>
</head>
<body>
<c:choose>
<c:when test="${num % 2 == 0}">
isEven: true
</c:when>
<c:otherwise>
isOdd: true
</c:otherwise>
</c:choose>
</body>
</html>
実行
$ mvn jetty:run
実行結果
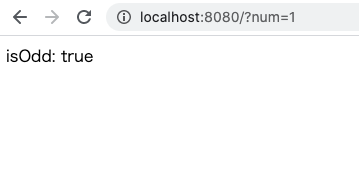
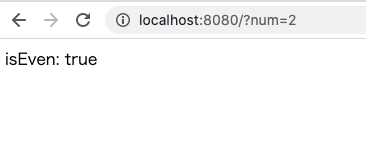